Python allows you to create and use range of values as arrays or lists in your scripts and programs. Generally, people use an integer as a step value between two consecutive values of range. For example, they may create a range 1, 2, 3, … 100. But sometimes you may need to use a decimal step value for range in Python. For example, 0, 0.1, 0.2, 0.3… 1.0. Typically, people use range() function to generate list ranges but it only works with integer step values. In this article, we will learn how to use decimal step value for range in Python.
How to Use Decimal Step Value for Range in Python
There are several ways to use decimal step value for range in Python. We will learn each of them one by one.
1. Using List Comprehension
If you don’t want to use a python library, you can also use list comprehensions to generate this list. Here is an example to generate a range of numbers, with a step value of 0.1. range() function can only generate a range of integers, not floating point numbers, so you need to multiply it with a floating point number to use a decimal step.
In this case, we simply multiple each of the range elements with the step value
>>> a = [x * 0.1 for x in range(0, 10)]
>>> print a
[0.0, 0.1, 0.2, 0.30000000000000004, 0.4, 0.5, 0.6000000000000001, 0.7000000000000001, 0.8, 0.9]
If you find that the multiplication with floating point numbers gives results with many decimal places, as shown above, then you can use round() function to round it as shown below.
>>> a = [round(x * 0.1,2) for x in range(0, 10)]
>>> a
[0.0, 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9]
If you already have a specific start and step value then you can modify the above code as shown below. In this case, we add range item * step value, to start value, in each iteration. Here we have a start value of 5 and step value of 0.2.
>>> start = 5
>>> step = 0.2
>>> a = [start + (x * step) for x in range(0, 10)]
>>> print a
[5.0, 5.2, 5.4, 5.6, 5.8, 6.0, 6.2, 6.4, 6.6, 6.8]
2. Using arange()
If you do not mind using a library for this purpose, then you can use Numpy library for this purpose. It contains arange() function that allows you to create a range of values using decimal step. Numpy is not available by default in Python. Here is the command to install Numpy.
$ pip install numpy
Here is the syntax of arange() function.
arange(starting, end, step)
In the above function, you need to provide the first item of list, the last item and the step for intermediate items.
Here is an example of arange() function.
>>> import numpy as np >>> np.arange(0.0, 1.0, 0.1) array([ 0.0 , 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9])
Please note, by default, arange() function does not include the endpoint, that is, 1 in the above example. To include it, add the step value to the endpoint in function call.
arange(starting, end + step, step)
Here is the code to include end point 1.0 in the list range.
>>> import numpy as np
>>> np.arange(0.0, 1.1, 0.1)
array([ 0.0 , 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9, 1.0])
3. Using linspace()
You can also use linspace function from Numpy library for this purpose. Its syntax is similar to that of arange().
linspace(start, end, num)
In the above function, linspace() function divides the difference between end and start into num equal parts. It also allows you to specify whether you want to include endpoints or not, unlike arange() function.
>>> np.linspace(0,1,11) array([ 0. , 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9, 1.0 ]) >>> np.linspace(0,1,10,endpoint=False) array([ 0. , 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9])
4. Using Generator Function
Often, you may need to create a large list range with many values. This can take up a lot of memory if you are not careful with how you generate such a list. Most of the above methods will take up a lot of space if your list is too long. If you need to create a really large range of values using decimal step, you can modify the above code to use a generator function, which consumes very less memory. Please note, the following code may look like a list comprehension but it uses round braces (…) to specify a generator function, and not square braces […] used for list comprehension.
xs = (x * 0.1 for x in range(0, 10)) for x in xs: print(x)
A generator function consumes less memory compared to list comprehensions since it does not generate and load the entire list in memory. Instead, it returns an iterator to the list, such that its value changes when referred during iteration.
Conclusion
In this article, we have learnt 4 different ways to use decimal step value in Python. You can use any of them as per your requirement. In many real world cases, we need list ranges with decimal step values. At such times, these solutions are very handy. In most cases, you can use list comprehensions to get the job done. If you are facing memory and space constraint, or if you need to create a large list, then you can go with generator function. If you are already using Numpy for data analysis, then you can choose arange() or linspace().
Also read:
How to Get Browser Viewport Dimensions in JS
How to Auto Resize TextArea to Fit Text
How to Render HTML in TextArea
JavaScript Object to String Without Quotes
Related posts:
Plot Graph from CSV Data Using Python Matplotlib
How to Count Repeated Characters in String in Python
How to Remove HTML Tags from CSV File in Python
How to Extract Numbers from String in Python
How to Convert PDF to Text in Python
How to Execute Stored Procedure in Python
Python: Reading & Writing to Same File
Random Password Generator in Python with Source Code
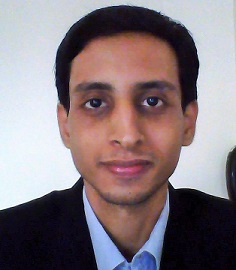
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.