Web browsers allow you to store data locally using Local Storage as well as Session Storage objects. In this article, we will learn how to store data in Local Storage using JavaScript.
How to Store Data in Local Storage in JavaScript
Local storage can be accessed using a global object localStorage and session storage can be accessed using global object sessionStorage. Both these objects offer getItem() and setItem() functions to retrieve and store data.
Let us say you have a test object as shown below.
var testObject = { 'one': 1, 'two': 2, 'three': 3 };
Here is the command to store data into local storage.
localStorage.setItem(variable, value);
Here is the command to store the above test object in local storage.
// Put the object into storage localStorage.setItem('testObject', JSON.stringify(testObject));
Here is the command to store data in session storage instead.
sessionStorage.setItem(variable, value);
Here is the command to store above test object in session storage.
// Put the object into storage sessionStorage.setItem('testObject', JSON.stringify(testObject));
Here is the command to retrieve the stored local object and display its value in console.
localStorage.getItem(variable);
Here is the command to retrieve value of stored object testobject.
// Retrieve the object from storage var retrievedObject = localStorage.getItem('testObject'); console.log('retrievedObject: ', JSON.parse(retrievedObject));
Here is the command to get data from session object.
sessionObject.getItem(variable)
Here is the command to get data from stored testobject.
// Retrieve the object from storage var retrievedObject = sessionStorage.getItem('testObject'); console.log('retrievedObject: ', JSON.parse(retrievedObject));
Please note, you can store any kind of data such as strings, numbers, arrays, not just objects, in local storage as well as session storage.
In this article, we have learnt how to store data in local storage.
Also read:
How to Display Local Storage Data in JavaScript
How to Enable, Disable & Install Yum Plugins
How to Delete Root Mails in Linux
How to Check if Element is Visible in Viewport
How to Check if Element is Hidden in JavaScript
Related posts:
How to Smooth Scroll on Clicking Links
How to Add Event to Dynamic Elements in JavaScript
How to Detect Invalid Date in JavaScript
How to Use Variable As Key in JavaScript Object
How to Measure Time Taken by JS Function to Execute
How to Get Duplicate Values in JS Array
How to Get Unique Values from Array in JavaScript
How to Remove Key from JavaScript Object
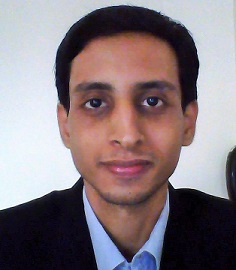
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.