Sometimes you may need to split string into array in Linux. You can easily do this using shell script. In this article, we will look at how to split string into array in shell script.
How to Split String into Array in Shell Script
Here are the steps to create shell script to split strings.
1. Create Shell Script
Open terminal and run the following command to create empty shell script.
$ sudo vi /home/split.sh
2. Add Shell Script
Add the following lines to your shell script.
#!/bin/sh string="Today is a beautiful day." IFS=', ' read -r -a array <<< "$string" echo 'printing elements' for element in "${array[@]}" do echo "$element" done
Save and close the file. In the above file, we first define shell script execution environment. Then we use the following command to split string into an array
IFS=', ' read -r -a array <<< "$string"
In the above command, we parse the string stored in $string variable based on characters present in IFS (Input Field Separator). Please note, $IFS will treat each character present in as a separate delimiter. In our case, it will treat comma and space as different separators, and not the sequence of comma+space.
Then loop through the array and display individual elements one by one. You may also display individual elements as
echo "${array[0]}" echo "${array[2]}" ...
If you want to display both index and value in each iteration of for loop, update it as shown.
for index in "${!array[@]}" do echo "$index ${array[index]}" done
3. Make Shell Script Executable
Run the following command to make your shell script executable.
$ sudo chmod +x /home/split.sh
4. Run Shell Script
You can run the shell script with the following command
$ sudo /home/split.sh Today is a beautiful day.
That’s it. As you can see it is very easy to split string into array in shell script. We have also seen a couple of useful modifications that may be made to your script, as per your requirement.
Also read:
How to Check if String Contains Substring in Bash
Shell Script to Remove Last N Characters from String
How to Find Unused IP Address in Network
How to Setup Email Alerts for Root Login
How to Extract IP Address from Log File
Related posts:
How to Loop Over Lines of File in Bash
How to Merge Folders & Directories in Linux
How to Configure DNS Nameserver in Ubuntu
How to Disable Auto Logout in Linux
How to Install .deb File in Ubuntu
How to Install Dpkg dependencies automatically
How to Repair Ubuntu 18.04 from USB
How to Copy File Permissions & Ownership from Another File in Linux
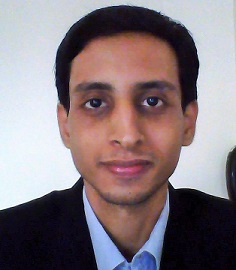
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.