Sometimes you may need to run same command multiple times in Linux. Instead of manually running the command, it is much better to automate it using different kinds of loops. In this article, we will learn how to run same command multiple times in Linux.
How to Run Same Command Multiple Times in Linux
We will some different ways to run same command multiple times in Linux.
1. Using for loop
Using for loop, you can easily run any command multiple times in Linux. Here is an example shell script to run shell command date one after the other in Linux.
#!/bin/bash for i in 1 2 3 4 5 do date done
In the above code, for loop runs 5 iterations. In each iteration, it calls date command.
You can save it as file multiple.sh and run it with the following command.
$ sudo chmod +x multiple.sh $ ./multiple.sh
But please note, these command calls to date will run one after the other immediately, without any delay. If you need to wait between successive function calls, you can use sleep function to wait for a specific amount of time.
#!/bin/bash for i in 1 2 3 4 5 do date sleep 1 done
In the above command, we wait for 1 second after each call to date command.
In each of the above case, the commands are run in the same shell. If you want to run each command in a separate shell, you can call it along with bash command, as shown below.
#!/bin/bash for i in 1 2 3 4 5 do bash -c "date" sleep 1 done
If you want to run long-running commands multiple time, without waiting for each call, or if you want to run command multiple times in parallel, you can try running them in separate shell as shown above.
2. Using while loop
Similarly, you can also use while loops to run command multiple times. Here is an example to run date command 5 times one after the other, using while loop.
#!/bin/bash i=0 while [ $i -lt 5 ] do date done
If you want to wait for a specific amount of time after each command call, use sleep command immediately after executing the command, in each iteration. Here is an example to wait for 1 second, after you run date command, in each iteration.
#!/bin/bash i=0 while [ $i -lt 5 ] do date sleep 1 done
You can also run these commands in separate shells if you want, using bash command as shown below.
#!/bin/bash i=0 while [ $i -lt 5 ] do bash -c "date" done
If you want to run long-running commands multiple time, without waiting for each call, or if you want to run command multiple times in parallel, you can try running them in separate shell as shown above.
In this article, we have learnt how to run Linux command multiple times. You can customize it as per your requirement. You can also use other tools like xargs command to run the same command multiple times, but it seems like an overkill, unless you want to pass different argument to each command call, and need to construct separate command statements for each argument. You can customize the above examples to even run a shell script multiple times, not just for command execution.
Also read:
Reload Bashrc Settings Without Logging In
How to Split File in Linux
Random Password Generator in Python
How to Generate Password in Linux
How to Use Wget to Download File Via Proxy
Related posts:
How to Get Current Directory of Bash Script
How To List All Services in Systemctl
How To Install Pip in Ubuntu Linux
How to Use Shell Variables in Awk Script
How to List Devices Connected to Network in Linux
How to Download File to Directory with Wget
How To Use Git Shallow Clone
How to Create Nested Directory in Linux with One Command
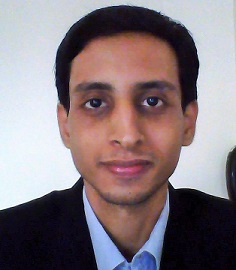
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.