Often you may need to increment or decrement variables in shell script. It is mostly done in loops as counter, but can be done elsewhere also. There are several simple ways to increment & decrement variable in shell script. We will learn them in detail, in this article.
How to Increment & Decrement Variable in Shell Script
Here are the different ways to increment & decrement variables in shell script. You can do this using double parenthesis ((…)) or $((…)) or with let command.
1. Using + and – operator
You can simply add/subtract the differential value to increment/decrement variable, using +/- operators. Here is an example to increment shell variable.
i=$((i+1)) ((i=i+1)) let "i=i+1"
Here is an example to decrement shell variable.
i=$((i-1)) ((i=i-1)) let "i=i-1"
You can use these operators to increment/decrement variables by any value you want.
Here is a simple example to use it in for loop.
for((i=0;i<5;i=i+1)) do echo i: $i done i:0 i:1 i:2 i:3 i:4
2. Using += and -= operators
In this expression you increment/decrement value of the left operand with the value in the right operand.
Here is an example to increment shell variable by 1.
((i+=1)) let "i+=1"
Here is an example to decrement shell variable by 1.
((i-=1)) let "i-=1"
Here is an example to use it in for loop where we increment shell variable by 2.
for((i=0;i<10;i+=2)) do echo i: $i done i:0 i:2 i:4 i:6 i:8
3. Using ++ and — operator
You can also use ++ and — operators to increment and decrement values respectively. But these operators will increment/decrement operators only 1, unlike above operators. You can use them as prefix or postfix operators meaning you can add them immediately before or after the operator.
Here is an example to increment shell variable using ++ operator.
#prefix increment ((++i)) let "++i" #postfix increment ((i++)) let "i++"
Here is an example to decrement shell variable using — operator.
#prefix increment ((--i)) let "--i" #postfix increment ((i--)) let "i--"
The main difference between prefix and postfix operators is that prefix operators increment/decrement the value first and only then return the updated value, while postfix operators increment/decrement the variable after returning the old value.
Here is an example to show the difference between prefix and postfix operators. First let us look at the prefix increment operator.
i=0 echo $((++i)) 1
Here is an example to show the usage of postfix increment operators.
i=0 echo $((i++)) 0
In this article, we have learnt how to increment & decrement shell variables. You can use these operators as per your requirement.
Also read:
How to Get Current Directory of Bash Script
Apache Commands Cheat Sheet
How to Clone Git Repository to Specific Folder
How to Switch Python Version in Ubuntu/Debian
How to Disable HTTP Methods in Apache
Related posts:
How to Downgrade RHEL/CentOS to Previous Minor Release
How to Restrict SSH Access to Specific IP Addresses
How to Run C Program in Linux
How to Reverse String in Python
How to Add Newline After Pattern in Vim
How to Install Virtualenv in Ubuntu
Sftp script to transfer files in Linux with password
How to Install Dpkg dependencies automatically
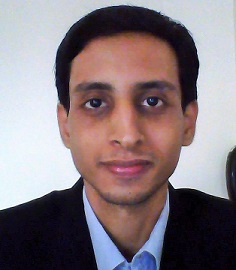
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.