JavaScript allows you to do tons of fun stuff on your website using arrays and other data structures. Sometimes you may need to extract a random element from an array. There is no out of the box function to do this. Of course, there are third-party JS libraries for this purpose. In this article, we will learn how to get random element from array in JavaScript.
How to Get Random Element from Array in JavaScript
The idea is to calculate a random array index and use it to get the array element. Let us say you have the following JS array.
var myArr = ['January', 'February', 'March'];
Every array element has an index by default. You can easily get an element using its index as array_name[index]. We just need to obtain a random number and use it as an index to get the element.
For this purpose, we will use the following function. It returns a random number between 0(inclusive) and 1 (exclusive).
Math.random();
We will multiply this with the number of elements in our array, myArr.length.
Math.random()*myArr.length
Since the above result may not be an integer we call Math.floor() function on it which will round down the number to the nearest integer.
Math.floor(Math.random() * myArr.length)
For example, if Math.random() returns 0.4, then the index will be Math.floor(0.4*3)= Math.floor(1.2)=1. You can use this index to get your array element.
myArr[Math.floor(Math.random() * myArr.length)];//February
If you have third party libraries like Underscore or Lodash, then you can use the sample() function to get a random element from array.
_.sample(['January', 'February', 'March']);
In this article, we have learnt how to get random element from array in JavaScript. You can use it as a standalone code or a part of another larger module. It can be used to pick random item from any kind of array. The key is to calculate a random integer whose value is less than the length of your array and use it to fetch the item.
Also read:
How to Simulate Keypress in JavaScript
How to Call Function Using Name in JavaScript
How to Detect Tab/Browser Closing in JavaScript
How to Include HTML in Another HTML File
How to Call Function When Page is Loaded in JavaScript
Related posts:
How to Test for Empty JavaScript Object
How to Get data-id attribute in jQuery / JavaScript
How to Split Array Into Chunks in JavaScript
How to Create Please Wait Loading Animation in jQuery
How to Scroll to Bottom of Div
How to Detect if Device is iOS
How to Validate Decimal Number in JavaScript
How to Detect Touchscreen Device in JavaScript
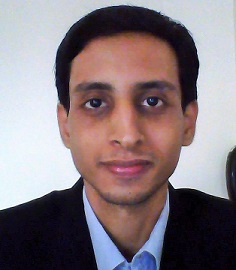
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.