Often you may need to add a ‘copy to clipboard’ feature to your web application or website. Previously, we had to use JS plugins for this purpose. But now browsers have started supporting this functionality natively. In this article, we will look at how to copy text to clipboard using plain javascript.
How to Copy to Clipboard in JavaScript
Here are the steps to copy text to clipboard using JavaScript.
1. Create HTML file
Open terminal and create blank HTML file.
$ sudo vi text.html
Add the following HTML file.
<!-- The text field --> <input type="text" value="Hello World" id="myInput"> <!-- The button used to copy the text --> <button onclick="copy_to_clipboard()">Copy to Clipboard</button>
The above HTML file adds a textbox and button. We have also added an event for clicking the button.
2. Add JavaScript
Add the following javascript code to your HTML file.
<script type="text/javascript"> function copy_to_clipboard() { /* Get the text field */ var Text = document.getElementById("myInput"); /* Select the text field */ Text.select(); Text.setSelectionRange(0, 99999); /* For mobile devices */ /* Copy the text inside the text field */ document.execCommand("copy"); /* Alert the copied text */ alert("Copied the text: " + Text.value); } </script>
In the above script we have added an event handler copy_to_clipboard() for click event fired when you click the button on HTML page. In that function, we first select our textbox. Then we use execCommand() to copy the text in textbox to clipboard. Finally, we display an alert box confirming that our text is copied. You can try pasting the copied in another document such as .txt file by simply typing Ctrl+P (Windows).
Please note, you can use the above function to copy text from other HTML elements also such as paragraphs or div, since we are using the id attribute of textbox to identify the element.
That’s it. As you can see, ever since browsers have started supporting copy to clipboard functionality, it has become very easy to add this feature on any web page, static or dynamic. You can easily modify and add it to your website/web application.
Also read:
How to Check Command Executed by User in Linux
How to View User Login History in Linux
How to Send Email in Python
How to Restrict SSH Access to Specific IP Address
How to Find Users Currently Logged in Linux
Related posts:
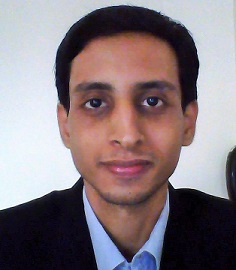
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.