jQuery is a popular JavaScript library that allows you to easily handle events on your web pages. But sometimes you may need to get ID of element that fired event in jQuery. This is typically needed if you need to do further processing with the ID of the element, in some other part of your code. In this article, we will learn how to do this.
How to Get ID of Element That Fired Event in jQuery
In jQuery event handlers, you can pass a variable to the handler function, for event object. That object’s target property refers to the element that fired the event. Here is an example to illustrate this. Let us say you have the following button on your page.
<button id='myButton'>click me</button>
Here is the code to handle the click event of this button.
$(document).ready(function() { $("#myButton").click(function(e) { alert(e.target.id); }); });
In the above code, we have attached the click() event to button with ID=myButton. In the event handler’s function we have used ‘e’ argument. This argument contains the event object that is passed to the handler function when button is clicked. This event object has property target (e.g. e.target) which refers to the element that fired the event. You can retrieve its ID by using the id property of the element (e.g. e.target.id).
Alternatively, you can also use this keyword which refers to the element that fired the event. Since this keyword is not an object, you need to refer to it as $(this), as shown below.
$(document).ready(function() { $("#myButton").click(function(event) { $(this).attr("id"); }); });
In the above code, $(this) refers to the element that fired the click event. We call attr() function to get the ID attribute of the element.
In this article, we have learnt how to get ID of element that fired event in jQuery. Of course, there are several other ways to do this but we have looked at a couple of simple ones.
Also read:
How to Get Property Values of JS Objects
How to Scroll to Bottom of Div
How to Remove Empty Element from JS Array
How to Check if Variable is Array in JS
How to Get Highlighted/Selected Text in JavaScript
Related posts:
How to Disable/Enable Input in jQuery
How to Change Element's Class in JavaScript
How to Check if JavaScript Object Property is Undefined
How to Test for Empty JavaScript Object
How to Get Subset of JS Object Properties
JavaScript Round to 2 Decimal Places
How to Add Days to Date in JavaScript
How to Replace Line Breaks With <br> in JavaScript
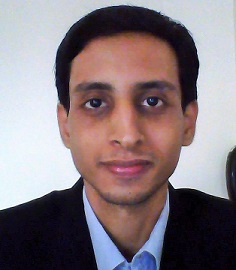
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.