Sometimes you may need to find the index of item in a Python List. You can easily do this using index() function available in every Python list, by default. But there are a few things to keep in mind, while using index() function. In this article, we cover how to find item index in Python list, and how to deal with certain edge cases.
How to Find Index of Item in List in Python
Here are the steps to find index of item in List in Python. Let us say you have the following list
>>> a=[1,2,3,4,5]
Here is the command to find index of element with value 3.
>>> a.index(3) 2
As you will see, the list items are indexed starting from 0. The first item is indexed 0, the second one is indexed 1 and so on. So it returns 2 as the index of value ‘3’.
You can even directly use it on list, instead of variable, as shown below.
>>> [1,2,3,4,5].index(3) 2
Please note, if the value does not exist, you will get a ValueError error. Here is an example when we try get index of value 6 which is not present in the list.
>>> a.index(6) Traceback (most recent call last): File "<pyshell#2>", line 1, in <module> a.index(6) ValueError: 6 is not in list
So if you are not sure whether the searched item exists in your list or not, then better wrap the index() command in a try…catch block to avoid uncaught errors in your application.
Also, here is the complete syntax of index() function.
list.index(x[, start[, end]])
In the above command, x is the item whose index is to be returned, start and end are optional arguments to limit the search to a specific subset of the list.
Please note, that index() function searches for the requested item in a linear manner, starting with the first item. So if your list is large with, say, thousands of items, then it can take a while for index() function to work. In such cases, it is better to limit the search sequence by mentioning start & end indexes. For example, let us say you have a large list of 1 million items
>>> a=[1,2,3,...,1000000]
Now if you were to get index of 999990 with the following command, it will take a really long time. This is because index function will have to start searching from the first item.
>>> a.index(999990)
But if you specify the start and end points as 999989 and 1000000 respectively, then the index function will just have to search 11 items and get the result quickly.
>>> a.index(999990,999989, 1000000)
Finally, it is important to note that the index() function only returns the index of the first occurrence of an item. If there are multiple occurrences of an item in a list, you will need to use a generator, list comprehension or some kind of loop to iterate through the list and get index of all occurrences of your item.
In this article, we have learnt how to get index of an item in python list.
Also read:
How to Redirect Using JavaScript
How to Show All MySQL Users
How to Upgrade All Python Packages with Pip
How to Create Python Function with Optional Arguments
How to Import Python Module Given Full Path
Related posts:
How to Check if String Matches Regular Expression
How to Setup Uwsgi with NGINX for Python
How to Check Version of Python Modules
How to Count Repeated Characters in String in Python
How to Check Python Package Path
How to Run Python Script in Django Project
How to Run Python Script in Apache Web Server
How to Flatten List of Tuples in Python
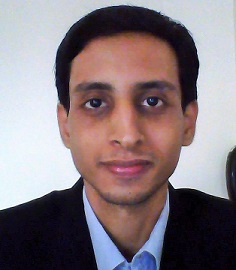
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.