Sometimes you may need to create subdomain programmatically or dynamically create subdomain for your website. Here is how to generate subdomains on the fly in PHP.
How to Generate Subdomains on the Fly in PHP (.htaccess)
If your clients require you to white-label your web application, they may ask you separate subdomains for themselves. In such cases, you will need to create subdomains on the fly, as more clients/customer sign up for your product/service.
Here are the steps to do it. In short, you need to enable your domain registrar to listen to all subdomains of your website. Then configure your Virtual Host to listen to all subdomains of your site. Finally, update PHP code to get subdomain from requested URL and display appropriate content.
1. Create WildCard DNS entry
Go to your domain registrar (e.g. Namecheap, GoDaddy, etc) website and create a wildcard entry.
Basically, create an A record with host value as * that points to your IP address. Only then your domain registrar will listen to all subdomain requests sent to your website.
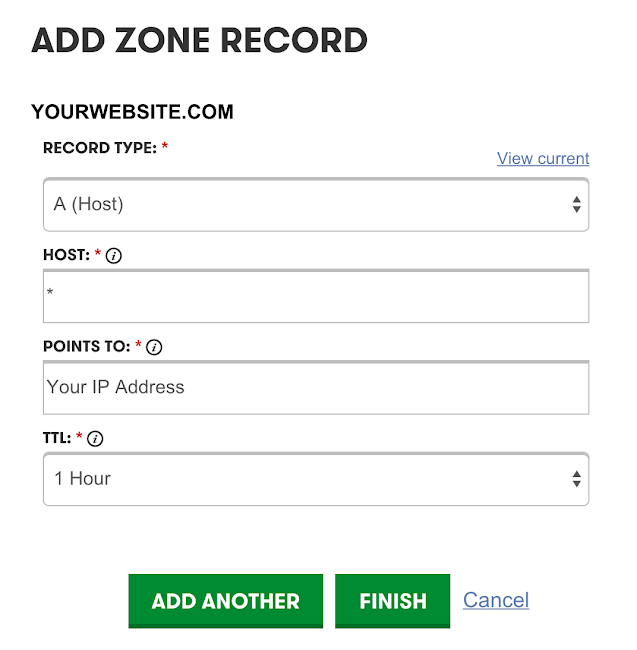
Similarly, create another A record like above, with Host value as @ and pointing to your IP address.
When you save this information, your A records will look something like the following
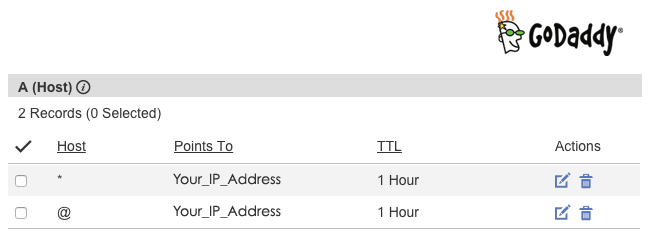
Also add a CNAME type of record just as above, with host value as www and points to ‘@’
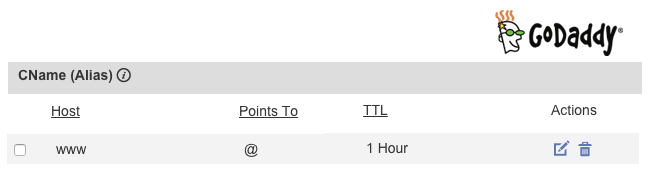
Also read : How to Remove index.php from URL in CodeIgniter
2. Update Virtual Host
Open your virtual host file of Apache server in a text editor. Please replace the path below with location of your virtual host file.
$ sudo vi /etc/apache2/sites-available/example.com.conf
It will look something like the following.
<virtualhost *:80=""> ServerName www.example.com UseCanonicalName Off ... </virtualhost>
Add ServerAlias Directive to your virtual host as shown below.
<virtualhost *:80="">
ServerName www.example.com
ServerAlias *.example.com
UseCanonicalName Off
...
</virtualhost>
Please note, you need to add * as the subdomain in ServerAlias directive. Only then your Apache server will listen to all subdomains requested on your website.
Also read : How to Check Which User is Using Apache
3. Update PHP code
Use PHP server variable to get subdomain. Here is the code snippet to do so.
preg_match('/([^.]+)\.example\.org/', $_SERVER['SERVER_NAME'], $matches); if(isset($matches[1])) { $subdomain = $matches[1]; } OR $subdomain = substr( $_SERVER['SERVER_NAME'], 0, strpos($_SERVER['SERVER_NAME'], '.') );
Once you have the subdomain, you can use it to display relevant content for each subdomain.
Here is an example that checks if subdomain == “test” and redirects to home page of test.example.com. Else it displays generic home page.
<?php $subdomain = substr( $_SERVER['SERVER_NAME'], 0, strpos($_SERVER['SERVER_NAME'], '.') ); ?> //HTML Code <!DOCTYPE html> <html> <head> <title>Home Page</title> </head> <body> <?php if($subdomain=="test") { ?> //Home Page for test.example.com <?php } else { ?> //Redirect to Generic home page <?php } ?> </body> </html>
That’s it. Now your web server will be able to handle all subdomains of your website. In this article, we have learnt how to serve subdomains on the fly in Apache.
Also read : How to Change Default Page in Apache
Related posts:
How to Enable PHP Error Reporting
How to Add Multiple Hosts in PHPMyAdmin
How to Run PHP Scripts Automatically
How to Downgrade PHP 7 to 5.x
How to Fix "Unable to find socket transport SSL" error in PHP
How to Prevent SQL Injection in PHP
How to Prevent Cross-Site Scripting in PHP/Apache
How to Enable PHP in Apache
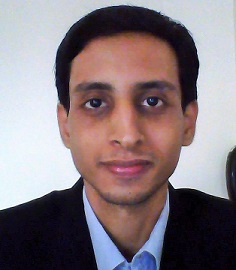
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.