Sometimes you may need to iterate over lines in file and display its contents. If you need to do this frequently, then it is advisable to create a shell script for this purpose. In this article, we will create a shell script to loop over lines of file in Bash. You can use this shell script for other shells also.
How to Loop Over Lines of File in Bash
Here are the steps to create a shell script to loop over lines of file.
1. Create empty shell script file
Open terminal and run the following command to create an empty shell script file.
$ sudo vi read_file.sh
2. Add Shell Script to Loop over Lines
Add the following lines to your shell script to loop over lines of file.
#!/bin/bash
while IFS="" read -r p || [ -n "$p" ]
do
printf '%s\n' "$p"
done < "$1"
Save and close the file. In the above code, we use $1 to take file path from command line argument. In our while loop we read the file line by line and output it using printf statement. This will ensure that whitespaces are not trimmed, backslashes are escaped, and last line is not skipped if it is missing a terminating linefeed.
If that is not a requirement for you, or if the above code does not work for you, then you may also use the following shell script instead. It also loops through the file line by line and echoes the output.
#!/bin/bash
while read p; do
echo "$p"
done < "$1"
3. Make Shell Script Executable
Run the following command to make the shell script executable.
$ sudo chmod +x read_file.sh
4. Test shell script
Test your shell script with different files as shown below
$ sudo ./read_file.sh file1.txt $ sudo ./read_file.sh /home/ubuntu/file3.txt
Please note, if you provide only filename above, then our shell script will look for the file in your present working directory.
If you want to run the shell script from another directory, just provide the full path to your .sh file as well as the full path to your file.
$ sudo /home/user1/read_file.sh /etc/data/file4.txt
That’s it. In this article, we have looked at how to loop through lines of a file using shell script.
Also read :
Shell Script to Tar Multiple Files & Directories
How to Create VirtualHost for Wildcard Subdomains in Apache
How to Check if Directory Exists Using Shell Script
Shell Script to Delete Files in Folders
How to Fix NGINX Upstream Closed Prematurely Error
Related posts:
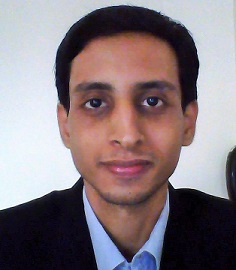
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.